It’s hard to build a Next.js app that reliably handles tasks that run longer than HTTP request timeouts. Use cases like these include:
- Processing large files in the background
- Handling webhook events in order
- Sending batches of emails on a schedule
The standard solution is to set up a separate service running a queueing system like BullMQ. This works, but it means deploying and maintaining additional infrastructure alongside your Next.js app.
DBOS offers a different approach. It’s an open-source TypeScript library that helps you durably execute long-running tasks directly in your Next.js app. It works by storing execution state in your database and if necessary, retrieving that data to resume execution after a failure. This ensures your tasks complete reliably, even if users disconnect, servers restart, or other interruptions occur.
How to Build a Durable Next.js App
Let’s build a simple Next.js app that runs a reliable background task. The app has two buttons: one that launches a background task and one that crashes the app. We’ll show that no matter how many times you crash the app, upon restart the background task will continue from where it left off.

Here’s the code for the background task. Each task runs ten steps, and each step sleeps for two seconds. The magic here is in the decorators: @DBOS.workflow() and @DBOS.step().

The decorators store the state of your program–what background tasks are running and how many steps they’ve completed–in a database:

This acts as a “save point” in your app. If it fails at any point, the background task can be restarted and picks up from the last stored checkpoint.
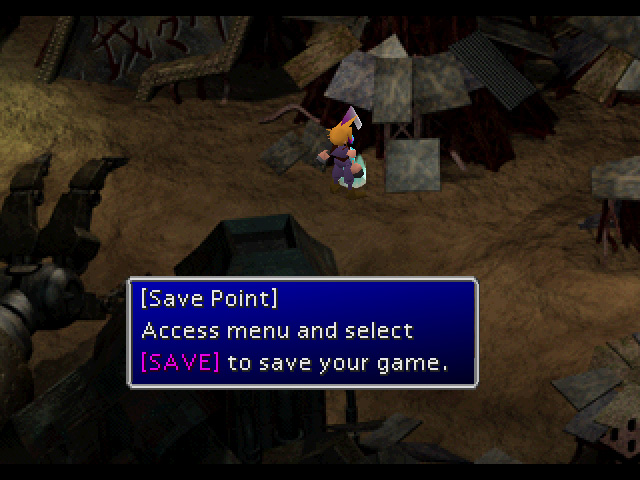
Because the background task is just a normal TypeScript function, you can start it from a Next.js server action. Here’s the code for the app’s server actions. The first action starts a background task, the second looks up the progress of a background task to display on the screen, and the third crashes the app–for demonstration purposes only 🙂.

You can call these server actions from buttons in your frontend code:
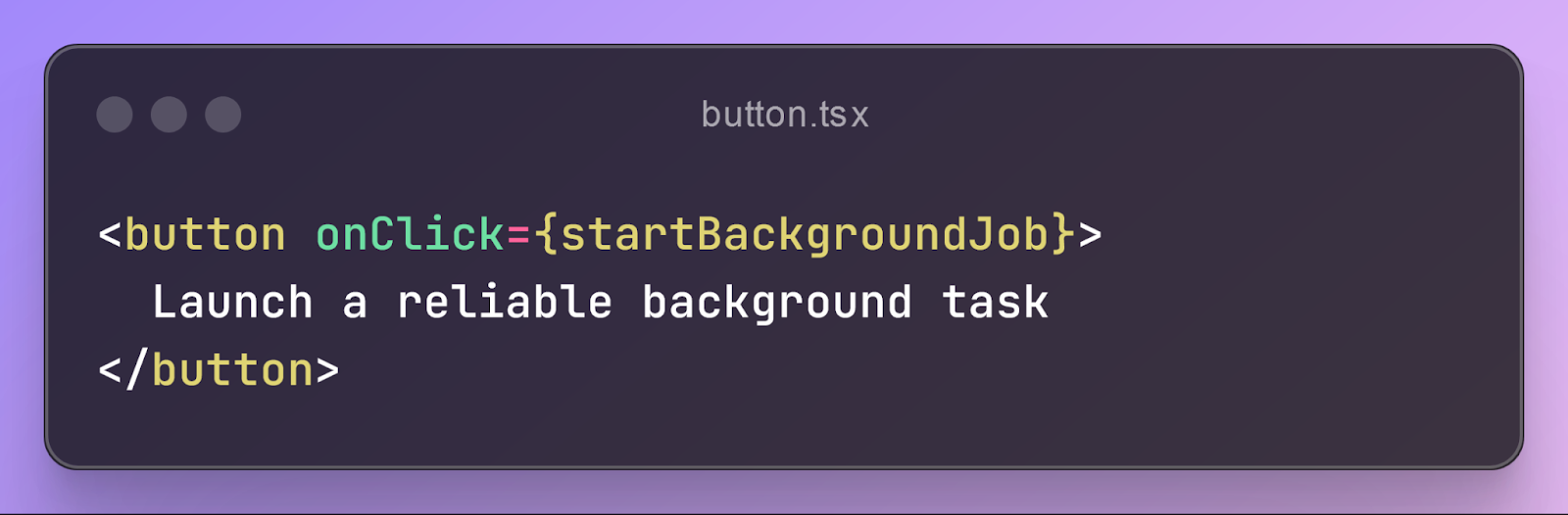
Run the Durable Next.js Example App Yourself
To try out this app yourself, initialize it from a template:
npx @dbos-inc/create -t dbos-nextjs-starter
Launch the development server:
npm run dev
Open localhost:3000 to see the app in action. Try launching a background task and crashing the app – you'll see the task resume exactly where it left off when you restart.
This example shows how to handle long-running tasks, but DBOS can help with other reliability challenges too:
- Running tasks in order with queues (like processing webhooks)
- Scheduling periodic jobs (like sending weekly emails)
- Automatically retrying failed operations